How To Migrate Your App And Protect It From Shutdowns Like Parse’s
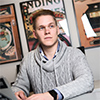
Editor’s Note: This article is written by Reinder de Vries, indie app developer at LearnAppMaking.com.
Oh no! Parse is shutting down… How do you move on?
On January 28th Parse announced it will be shutting down its service. They’re winding down the done-for-you Backend-as-a-Service, fully retiring it on January 28, 2017.
That’s bad news for over 500.000 developers who relied on the service since 2011. Like Jameson argued in his recent blog post, developers are feeling betrayed in their trust.
Parse was acquired in 2013 by Facebook for $ 85 million and now the “Big Five” monarch cold-bloodedly pulls the plug on the backbone of many developer’s apps.
Back in 2013 Parse was a risky acquisition, which Facebook needed to get a foothold in the mobile market. Facebook’s recent earnings report shows a growth in mobile advertising revenue of 52%, comparing Q4 of 2014 and 2015, which clearly shows they’ve landed in mobile.
With the rise in competition of cloud services by the likes of Amazon, Microsoft and Google, you could argue Facebook doesn’t need Parse anymore. From a business perspective I couldn’t agree more with them, but from a developer’s perspective it’s an awful move to make.
Or is it?
Parse’s Shutdown Is An Opportunity For App Developers
Before I built apps, I built websites. Lots and lots of websites. I learned all the necessary skills: HTML, CSS, JavaScript, PHP and MySQL. The quality and elegance of my code back then would make your skin crawl, but through trial and error I eventually mastered delivering quality websites.
Throughout the years I kept my craft up-to-date by building the occasional web app. Newer and more advanced tools became available and my job required I use them. I’ve transitioned from jQuery to Angular to React, touched on too many JavaScript frameworks in between, saw the rise of package managers and GitHub (SourceForge, anyone?), the switch from PHP 4 to PHP 5 to PHP 5.6 and the upcoming PHP 7. My MySQL tables exploded, so I switched to Elasticsearch and Mongo.
A while back I wanted to automate email newsletter signups and TestFlight beta invites. If you’d subscribe to the newsletter and confirmed your subscription, the workflow would automatically add you to my TestFlight pool of beta testers. I knew about the awesome FastLane toolset, and its boarding component, and figured boarding‘s HTML form must have a POST URL endpoint I could hijack. Essentially, I’d catch the subscriber’s email address from my form and input it into the boarding form. Boarding would take care of the rest, like adding the subscriber to the TestFlight beta tester pool.
There was only one problem: the boarding form had CSRF protection, which meant it expected a unique token to be included in every request. Those tokens are created server-side and in my workflow I didn’t have access to them.
I had access to boarding‘s source code, so technically I could solve the problem by disabling the CSRF protection. There was only one caveat: the source code was in Ruby, and I haven’t seen a single line of Ruby in my entire life (OK, maybe one), let alone have written one.
Thanks to my extensive background in back-end programming, I’m not afraid of getting my hands dirty with technology I don’t know. Years of tinkering and making mistakes gave me a perfect understanding of how the web works. Instead of shying away from a black box, I dug deeper.
I saw libraries I’d never heard of, with even stranger names (is this a thing?), and finally found the file that governed the CSRF protection feature. Ultimately, turning it off simply meant “nulling” a controller.
The Back-End Black Box
Almost every successful app uses some sort of back-end service to connect app users to each other, and persist user data on a server.
Many developers I meet and coach don’t have a solid understanding of back-end programming. Once they set up their Parse configuration in the overly simple Dashboard, write a query, they just assume their data is “out there”. Why would you bother finding out what happens in the cloud?
I often explain Parse as “a spreadsheet for your app”. It’s true: the Parse Dashboard isn’t more complicated than Google Spreadsheets, yet it powers the entire back-end of your app. You don’t even have to write queries for data, instead you use convenience methods like:
PFQuery
(className:
"Tweet"
).whereKey(
"user"
, currentUser);
It’s perhaps also Parse’s fault. Their PFQueryTableViewController
is so incredibly easy to use, but pretty dangerous: it directly couples back-end code with your app’s view controller (which is already a ViewModel). Even if you wanted to switch from Parse to Firebase, your code is so tightly coupled you have to rewrite your entire app before you can migrate.
Parse’s PFObject
class has mapping built in, so you can work with string data as native Swift String
objects, while in fact the Parse SDK turns that into data it can send over the internet. If you pass around PFObject
instances in your user-facing code, you’re going to have a hard time replacing that with a new Backend-as-a-Service SDK.
If you would have built your own back-end from scratch, you would have known for instance that true
(a boolean) is sometimes turned into "true"
(a string) when encoded as JSON. If that hits your app, you’re suddenly working with an instance of String
instead of the Bool
you expected. Fortunately Swift has an incredible type system, but an if let
optional binding statement only protects against a type mismatch — it doesn’t resolve it!
So how’s this Parse shutdown good for you? It’s time to start tinkering again, to dig deeper into the black box and to protect your code against shutdowns like Parse’s.
3 Ways To Make Your App Shutdown Proof
Next time you build an app, keep these three heuristics in mind. Instead of relying for 100% on one provider whose service is vital to the continuity and success of your app, you can protect yourself against shutdowns.
1. Make a list of alternatives
Parse has a couple of similar providers, like Firebase. Perhaps you didn’t even know Firebase existed until you Googled for alternatives in reaction to Parse’s shutdown.
The cool thing is: most of these services know you’re looking for them. If you Google “parse shutdown” or “parse migration”, you’re hit with multiple highly relevant Google AdWords ads that tell you to migrate over to their service. Don’t do it!
Instead, make a list of alternatives. Just make yourself comfortable with what’s out there. It’s never a bad idea to have a list of providers you rely on, and their alternatives. Just like a supermarket has a list of alternative milk suppliers in case their main provider goes out of business, you should keep a list of companies you do business with.
You can of course also use the list of alternatives to do some proper research about the technologies and SDKs you’re going to use. I didn’t know OneSignal existed until I started looking for a free push provider, to replace Urban Airship, because I found it too expensive.
2. Decouple your code
Regardless of how dull it may sound: Model-View-Controller still has a point. We’re getting used to coding Model-View-Whatever, but the principle of MVC remains: your Controller shouldn’t configure Views, and your View shouldn’t manipulate a Model.
Cocoa Touch makes it hard to not use MVVM (Model-View-ViewModel), but that doesn’t mean you should tightly couple your Model code in your View Controller code.
In programming theory we’ve got two principles: coupling and encapsulation. Working with two tightly coupled classes means ClassA makes a lot of assumptions about the inner functionality of ClassB.
Imagine a car and a driver. The driver sits in the front of the car, because the steering column is directly connected to the engine. What if the driver suddenly decided to sit in the back, in order to drive the car? Then the driving doesn’t work. Of course, such a situation never happens in real life, but it happens in your code all the time.
A protection against tight coupling is encapsulation. This means every class has a strictly defined set of methods, such as speedUp()
and steerLeft()
. Instead of letting the driver inject more gas into the combustion chamber, relying on the engine being of the combustible kind, you simply let the driver call speedUp()
. This way the driver doesn’t have intricate knowledge about the workings of the engine, but instead calls upon a function he knows is safe.
When the engine gets replaced by an electric one, which doesn’t have a combustion chamber, you don’t have to change the driver’s code: he relies on a speedUp()
method to be present, instead of relying on a injectGasMixture()
, gasMixture
and sparkTimer
.
If you find the principle of decoupling and encapsulation hard to grasp, think of it as responsibilities. Whose responsibility is it to drive the car? The driver’s. Who’s responsibility is it to make the car able to move forward? The engine’s.
When you’re coding your back-end enabled app, make sure you clearly define its components and their responsibilities. Create a class you only use as an intermediary between back-end code and front-end code. The front-end code is based on your own native classes, like Car
, and the back-end code has intricate knowledge about the back-end SDK you use, and works with classes like PFObject
.
It’s the intermediary’s job to communicate between the front-end and the back-end. In case your back-end code becomes obsolete, you can replace the intermediary with code that understands your new back-end SDK, without rewriting the front-end code as well.
3. Use the Adapter Pattern
The third and last heuristic ties into the principle of loosely coupled code. The Adapter Pattern, older than the internet itself, is an Object-Oriented Programming pattern. It’s a set of programming design rules you can use to make two incompatible classes compatible with each other.
It’s similar to making a “wrapper”, but instead of just exposing the interface of one resource (like wrapping a REST API) it also mediates between two incompatible interfaces by defining a set of rules. In many programming languages these rules are called interfaces (Java and PHP), in Swift and Objective-C they’re called protocols.
You do know Swift’s generics, right? If it swims like a duck and quacks like a duck, it must be a duck. The Adapter Pattern has a similar mechanism. Instead of integrating ClassA and ClassB tightly with each other, by making direct calls from A to B, you build an adaptor class around ClassA.
The adapter class has intricate knowledge about how ClassA works. The adapter class also conforms to a protocol. The protocol says: “You can be sure I have methods X, Y and Z”.
ClassB doesn’t know ClassA. It only knows it wants to work with an instance that conforms to the adapter’s protocol. It doesn’t care if the instance is a duck, as long as it swims and quacks like a duck.
What’s the benefit of working with an adapter? Well, in case your original ClassA code becomes obsolete, you can just find another class (i.e. another Parse alternative) and write an adapter for it. As long as this adapter conforms to the protocol, the rules you defined earlier, ClassB still works.
In reality, you would write an adapter (MyTwitterAdapter
) that has a method with this signature:
class
MyTwitterAdapter
:
AnyTwitterServiceProtocol
{
func
getTweetsWithUser(user:
User
, withPage page:
Int
= 0)
{
...
The protocol (AnyTwitterServiceProtocol
) contains the exact same method signature, but it doesn’t have any logic or method implementation. It just sets the rules.
protocol
AnyTwitterServiceProtocol
{
func
getTweetsWithUser(user:
User
, withPage page:
Int
= 0);
...
The front-end code (MyTweetsViewController
) has a variable twitter
of an undefined type, which it got from the “adapter factory”. This factory ensures you only have to replace class names once (or twice) if you change adapters.
If you’d strictly define twitter
to be of type MyTwitterAdapter
, you would still tightly couple your code, most likely in more than one place. Then, if you replace your adapter for a new one, you still have to update a lot of code.
Once you’ve got this set up, you can test if the twitter
instance you’re working with conforms to the AnyTwitterServiceProtocol
.
if
let
_twitter = twitter
as
?
AnyTwitterServiceProtocol
{
// Yes, it conforms
}
Your front-end code doesn’t care anymore whether twitter
is of type Car
, FooBar
or Facebook
, as long as it conforms to AnyTwitterServiceProtocol
. It simply wants to know if the instance has a method called getTweetsWithUser:withPage:
.
Note: in case you do want to strictly define the type of twitter
, you can use a protocol as a type, and you can also work with generics.
What’s Next?
Now that you’ve learned that you should improve your business operations and your reliance on suppliers, and protect your code against sudden changes, you can figure out how to do that.
Are you going to switch to Firebase? You should know that Firebase is owned by “Big Five” Google, and although Parse’s shutdown is no harbinger of more shutdowns, solely relying on a new provider leaves you equaly vulnerable as you were. Unless you protect your code, of course.
A good exercise would be to learn about back-end programming. Parse has released an excellent replacement product, Parse Server, which you can run on your own server (as long as it can run NodeJS and MongoDB). In the following code guide I’ll show you how to migrate a simple app from Parse to Parse Server, with Heroku and MongoLab.
Continue: Guide: How To Migrate Your Parse App To Parse Server With Heroku And MongoLab
Another relatively easy exercise is to migrate your Push Notifications to two services instead of one. Implementing the client-side SDK of a push provider is relatively easy, usually you only have to send over the unique push ID and set up a segment.
Use the Adapter Pattern to create a unified interface your front-end code can talk to. Decouple the actual adapter from the front-end code by defining a protocol. Pick a second Push Notifications provider (like Urban Airship, Mixpanel, Pusher or PushOver) and write a second adapter. Make sure it conforms to the protocol, and then simulate a service breakdown in which you have to deploy your new adapter. Does it work?
Conclusion
Don’t be afraid to go deep into the black box. It’s not a bad idea to write your own back-end REST API, just for the fun and experience of it. You’ll learn a lot.
Did you know Crashlytics is owned by Twitter? Anything could shutdown… Make sure you know what Crashlytics does for you, and how it works. It’s the only way to build a stable, reliable and long-term profitable business.
Down the rabbit hole you go.
About the author
Reinder de Vries is an indie app maker who teaches aspiring app developers and marketers how to build their own apps at LearnAppMaking.com. He has developed 50+ apps and his code is used by millions of users all over the globe. When he’s not coding, he enjoys strong espresso and traveling.